React 组件通信 01
组件之间是存在嵌套关系的,如果我们将一个程序的所有逻辑都放在一个组件中。那么这个组件将会变得臃肿不堪并且难以维护,所以组件化的思想就是对组件进行拆分,再将这些组件嵌套在一起,最终形成应用程序。
如图:
- app 组件是 Header Main Footer 组件的父组件
- Main 组件是 Banner ProductList 组件的父组件
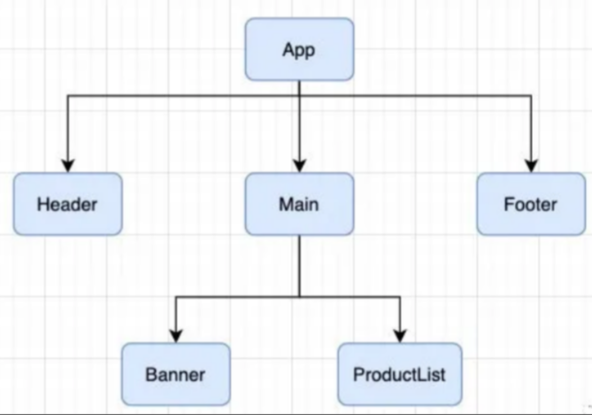
在开发过程中就涉及到了组件之间的通信:父子组件通信,兄弟组件通信,祖孙组件通信等等。
父传子通信
父子组件之间的通信是通过 props 来完成的
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| function ChildCpn(props) { const { name, age, height } = props;
return <h2>{name + age + height}</h2>; }
export default class App extends Component { render() { return ( <div> <ChildCpn name="zhangsan" age="18" height="1.88" /> <ChildCpn name="lisi" age="20" height="1.98" /> </div> ); } }
|
属性验证:
对于传递给子组件的数据,有时候我们可能希望进行数据格式验证
1 2 3 4 5 6 7 8 9 10
| import PropTypes from "prop-types";
ChildCpn.propTypes = { name: PropTypes.string, age: PropTypes.nimber, height: PropTypes.number, names: PropTypes.array, };
|
对数据进行验证时,如果数据类型不符,则会报错
如果父组件什么都没传递,我们可以给数据设置默认值
1 2 3 4 5 6
| ChildCpn.defaultProps = { name: "React", age: 30, height: 1.88, names: ["aaa", "bbb", "ccc"], };
|
子传父通信
只要让父组件给子组件传递一个绑定了自身上下文的回调函数,那么在子组件中调用这个函数的时候,就可以将想要交给父组件的数据以函数参数的形式传递出去,这样就形成了从子组件到父组件的流动
具体步骤
- 父组件将回调函数通过 props 传递给子组件
- 子组件把父组件需要的数据作为回调函数的参数传递
- 子组件调用该回调函数
简单的计数器案例:
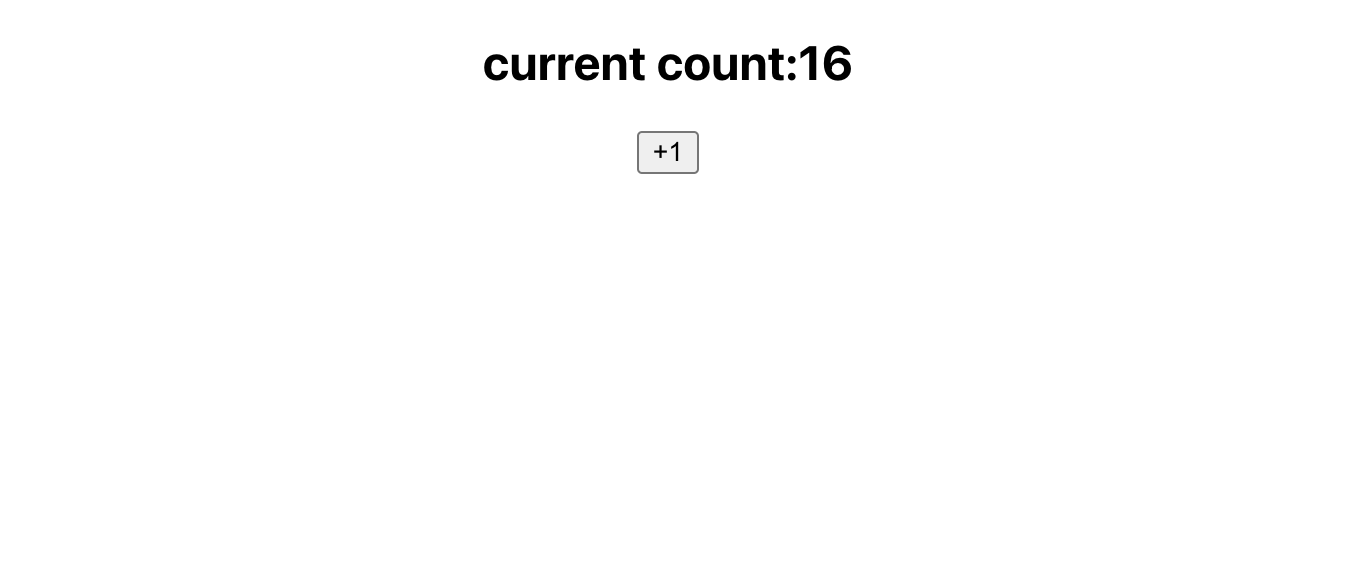
代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| import React, { useState } from "react";
const CounterButton = (props) => { const { handleClick } = props; return ( <div> <button onClick={handleClick}>+1</button> </div> ); };
const Counter = () => { const [counter, setCounter] = useState(0); const increment = () => { setCounter(counter + 1); }; return ( <div> <h2>current count:{counter}</h2> <CounterButton handleClick={increment} /> </div> ); };
export default Counter;
|
在父组件中定义了 increment 方法,当点击子组件按钮的时候就会触发父组件的方法,并执行该方法,实现数据加一